3D场景中如何控制物体的移动
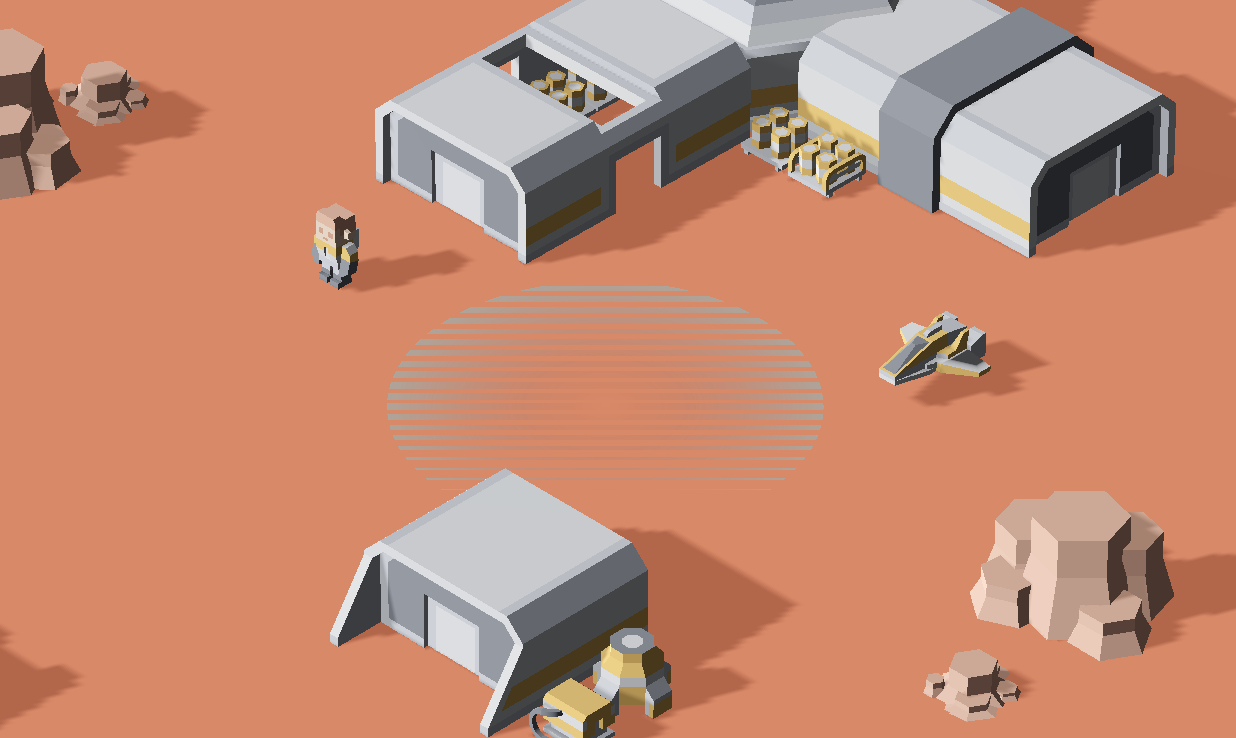
场景搭建
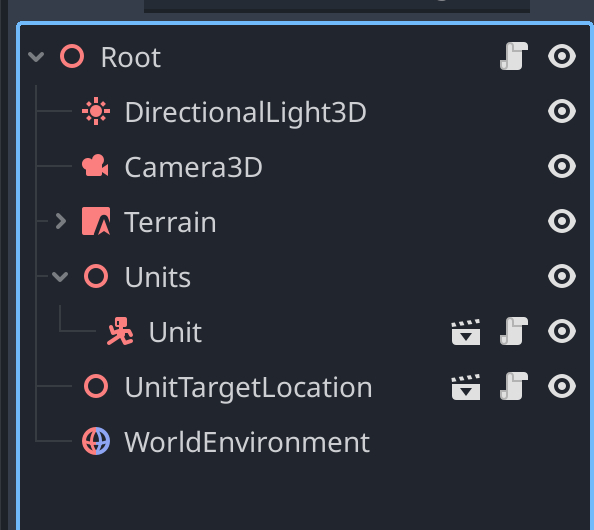
基础3D场景的搭建
navigaion的创建
创建Navigation3D
节点,然后将所有的3D场景放在这个节点下,在该节点中创建NavigationMesh
,点击烘培导航地图
如果新增了3D物体,则需要重新烘培,会自动创建移动路径。只是需要注意移动的物体以及Navigation3D
节点中Agent属性要与移动物体的碰撞体积的设置一致,否则会穿模
控制人物的移动
脚本如下
主脚本GameManager.gd,监听鼠标右键事件,然后将Unit移动到目标位置
extends Node3D
const _GROUND_MASK = 2
@onready var _camera: Camera3D = $Camera3D
@onready var _unit_target_location: UnitTargetLocation = $UnitTargetLocation
@onready var _unit: Unit = $Units/Unit
func _input(event):
if event is InputEventMouseButton and event.pressed and event.button_index == MOUSE_BUTTON_RIGHT:
var origin = _camera.project_ray_origin(event.position)
var direction = _camera.project_ray_normal(event.position)
var end = origin + direction * 1000
var state = get_world_3d().direct_space_state
var query_params = PhysicsRayQueryParameters3D.new()
query_params.from = origin
query_params.to = end
query_params.collision_mask = _GROUND_MASK
var intersection = state.intersect_ray(query_params)
if intersection.size() > 0:
# we hit the ground
var pos = intersection["position"]
_unit_target_location.click(pos)
_unit.move_to(pos)
物体移动控制脚本Unit.gd
class_name Unit
extends CharacterBody3D
@onready var _agent: NavigationAgent3D = $NavigationAgent3D
var movement_target: Vector3:
get:
return _agent.target_position
set(value):
_agent.target_position = value
func _ready():
# These values need to be adjusted for the actor's speed
# and the navigation layout.
_agent.path_desired_distance = 0.5
_agent.target_desired_distance = 0.5
# Make sure to not await during _ready.
call_deferred("actor_setup")
func actor_setup() -> void:
await get_tree().physics_frame
movement_target = global_position
func move_to(pos: Vector3) -> void:
movement_target = pos
func _physics_process(delta: float) -> void:
if _agent.is_navigation_finished():
return
var current_agent_position = global_position
var next_path_position = _agent.get_next_path_position()
var new_velocity = (next_path_position - current_agent_position).normalized()
new_velocity *= _agent.max_speed
velocity = new_velocity
move_and_slide()
if (next_path_position - current_agent_position).length_squared() > 0.01:
look_at(next_path_position, Vector3.UP)
目标点光标控制脚本
class_name UnitTargetLocation
extends Node3D
@onready var _anim: AnimationPlayer = $AnimationPlayer
func _ready():
# initialize to transparent colors everywhere
var m: ShaderMaterial = $Mesh.get_active_material(0)
m.set_shader_parameter("radius", 0.01)
func click(position: Vector3):
global_position = position + Vector3.UP * 0.02
_anim.play("UnitTargetLocation")